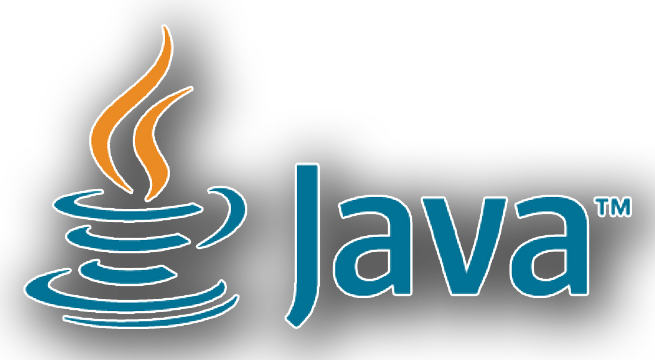
Introduction to Java
Keywords
Java Keywords
abstract |
continue |
for |
new |
switch |
assert |
default |
goto |
package |
synchronized |
break |
double |
implements |
protected |
throw |
byte |
else |
import |
public |
throws |
case |
enum |
instanceof |
return |
transient |
catch |
extends |
int |
short |
try |
char |
final |
interface |
static |
void |
class |
finally |
lomg |
stritfp |
volatile |
const |
float |
native |
super |
while |
Arithmetic Operators
Just like mathematics, Java has several arithmetic operators that you can use for computation. They are:
Java Arithmetic Operators
Operator |
Meaning |
Example |
Outcome |
+ |
Add |
x, y = 3, 4
z = x + y |
z is equal to 7 |
- |
Subtract |
x, y = 3, 4
z = x - y; |
z is equal to -1 |
* |
Multiply |
x, y = 3, 4
z = x * y |
z is equal to 12 |
/ |
Divide |
x, y = 6, 2
z = x / y |
z is equal to 3 |
% |
Modulus |
x, y = 5, 3
z = x % y |
z is equal to 2 |
** |
Exponent |
x, y = 2, 3
z = x ** y |
z is equal to 8 |
// |
Floor Division |
x, y = 20, 12
z = x // y |
z is equal to 1 |
There are a couple of these operators that need some explanation. First the modulus operator will return the remainder for a division problem.
- 10 % 3 results in 1 be case 10 / 3 = 3 with a remainder of 1
- 12 % 7 results in 5 Because 12 / 7 = 1 with a remainder of 5
The floor division operator gives a division of a number where the numbers after the decimal point are truncated.
- 15 // 4 is 3 because 4 goes into 15 three times; the remainder is truncated.
Escape Characters
The following table is a complete list of escape characters that can be used to format strings.
Java Escape Characters
Character |
Description |
\a |
Bell |
\b |
Backspace |
\Cx |
Control-x |
\e |
Escape |
\f |
Formfeed |
\M-\C-x |
Meta-Control-x |
\n |
Newline |
\r |
Carriage Return |
\s |
Space |
\t |
Tab |
\v |
Vertical Tab |
\x |
Character x |
\xnn |
Hexadecimal notation. Where n is in the range of 0-9, a-f or A-F |
Bitwise Operators
Bitwise operators in Java
Operator |
Meaning |
Example |
& |
Bitwise AND |
x& y = 0 (0000 0000 ) |
| |
Bitwise OR |
x | y = 14 (0000 1110 ) |
~ |
Bitwise NOT |
~x = -11 (1111 0101 ) |
^ |
Bitwise XOR |
x ^ y = 14 (0000 1110 ) |
>> |
Bitwise right shift |
x>> 2 = 2 (0000 0010 ) |
<< |
Bitwise left shift |
x<< 2 = 40 (0010 1000 ) |